If you need to verify whether an email is real, active, or safe to use, Laravel’s built-in validation rules may not be enough.
Today, we will show you how to do Laravel email validation step by step, from using the validation method to creating custom validation rules. But if you’re looking for an easier way – one that checks more than just the format – you’ll also find a better alternative that keeps invalid, fake, or risky emails off your list.
How to do Laravel email validation?
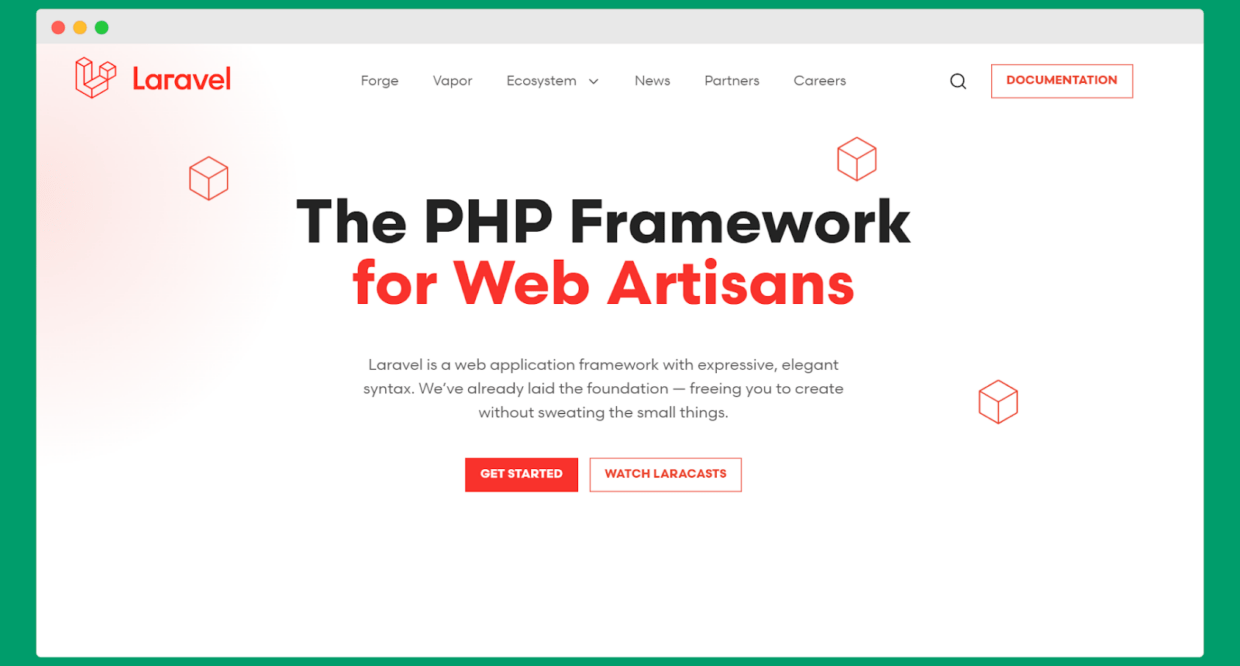
Laravel provides built-in email validation rules, along with the ability to create custom validation rules to fit specific needs. We will walk you through setting up Laravel email validation, handling validation errors, and implementing DNS and RFC validation.
#1 Validating email in Laravel using the validate method
Laravel’s validate method, available in the Illuminate\Http\Request class, makes it easy to validate incoming HTTP requests. The following example demonstrates basic email validation rules inside a controller method.
What these rules do:
- required – The field can’t be empty.
- email:rfc,dns – Checks if the email follows RFC standards and verifies the domain using DNS records.
If validation fails, Laravel automatically redirects users and flashes error messages in the session.
#2 Using form request validation for cleaner code
A form request class helps keep validation logic separate from the controller. Run this command:
Then, update the new class inside app/Http/Requests/:
Now, update your controller to use it:
This approach makes your code cleaner and reusable.
#3 Customizing validation error messages
Laravel has default error messages, but you can customize them in resources/lang/en/validation.php:
Or inside your form request class:
#4 Creating custom email validation rules
If you need something more advanced, create a custom validation rule:
Update app/Rules/ValidEmail.php:
Apply it inside a controller:
This allows for custom error handling and advanced validation.
#5 Handling validation errors
If validation fails, Laravel automatically returns errors. To show messages in Blade:
For API requests, Laravel sends a 422 response with errors:
#6 Advanced email validation: DNS & MX records
To validate an email’s domain, use DNS validation:
- rfc – Ensures the email follows RFC 5322.
- dns – Checks if the domain has MX records (i.e., it can receive emails).
For custom DNS validation, create a rule:
Update app/Rules/DnsValidator.php:
Apply it inside a controller:
#7 Validating email in JSON API requests
For API requests, return JSON responses instead of redirects:
Laravel automatically returns:
To handle validation manually:
Why is validating emails with Laravel not ideal?
Laravel email verification has its limitations. It only checks if an email follows the correct format – it doesn’t verify if the email is real, active, or safe to use. Here’s why relying solely on Laravel’s built-in validation rules might not be the best choice.
It only checks the address format
The validate method provided by Laravel ensures that an email looks correct, but it doesn’t confirm if the address exists. The validation rules focus on structure, not deliverability. This means invalid emails, typos, or even fake addresses can still pass through.
It does not cover catch-all addresses or spam traps
Laravel’s validation method doesn’t detect catch-all email addresses, which accept all incoming messages—valid or not. It also can’t filter out spam traps, which email providers use to catch senders using outdated or purchased email lists. Without these checks, your email list could fill up with risky addresses.
It requires coding knowledge to implement
Setting up Laravel email validation requires working with a PHP file, using the PHP namespace app structure, and writing custom validation rules. If you’re uncomfortable with Laravel’s syntax, applying your validation rules might be challenging. Custom error messages also need to be manually configured using the validation method.
It cannot be automated
Laravel doesn’t provide built-in automation for email validation beyond form submissions. You would need to integrate external APIs or write custom validation rules to validate emails dynamically. Even with a custom error message system, manually handling validation failure can slow down development.
The better alternative to validating emails with Laravel
While Laravel’s email validation rules help with basic format checks, they aren’t enough for maintaining a clean and reliable email list. To verify if an email is valid, consider using a tool that ensures your emails reach real users without the risk of bounces or spam traps.
Bouncer is a great solution here.
How Bouncer verifies emails:
You can upload your email address list and let Bouncer do the rest:
- Syntax and format check – Ensures the email follows proper structure (e.g., name@example.com).
- Domain and MX record check – Verifies if the domain exists and has mail servers configured to receive emails.
- SMTP validation – Bouncer connects to the recipient’s mail server (without sending an email) to check if the inbox exists and if messages can be received.
- Catch-all and spam trap detection – Identifies risky domains that accept all emails (even invalid ones) or trap spam senders.
- Toxicity check – Flags potentially harmful emails like disposable, temporary, or known fraudulent addresses.
Bouncer: what Laravel’s email validation doesn’t cover
Bouncer offers what Laravel’s built-in validation rules don’t – real verification that goes beyond just checking the format.
Bouncer confirms if an email is valid
Laravel applications rely on syntax-based validation. The validate method provided by Laravel only checks if an email follows the correct structure. It doesn’t check if an inbox exists or if the email is reachable. Bouncer, on the other hand, verifies valid email addresses by connecting with mail servers, ensuring every address is truly active.
It catches invalid, malicious, and toxic emails
Laravel version updates have improved email validation, but they still don’t detect spam traps, fraudulent addresses, or catch-all domains. Bouncer’s Bouncer Shield feature blocks invalid and malicious emails instantly, protecting your list from risky contacts that could hurt your sender’s reputation.
Automated verification without coding
Writing a custom validation rule in a PHP file means manually configuring the logic, handling validation failure, and defining a custom error message. Bouncer eliminates this extra work with an easy-to-use platform and API, making bulk email verification simple – no manual coding required.
Checks beyond format with deliverability insights
While Laravel validation rules can flag missing ”@” symbols, they don’t offer insights into email deliverability. Bouncer provides a Deliverability Kit that tests inbox placement, checks email authentication, and monitors blacklists to ensure your messages reach real users.
Bulk and real-time verification for better accuracy
Laravel applications process one email at a time using validation methods within controllers. Bouncer, however, supports bulk verification, letting you clean thousands of email addresses in one request. Its real-time API ensures new emails are verified instantly, preventing invalid signups and reducing bounce rates.
👉 Check out how to validate email addresses step by step, no coding.
Why choose Bouncer over Laravel’s validation?
- Laravel’s validation method ensures an email looks valid. Bouncer confirms if it actually works.
- Laravel requires custom validation rules for advanced checks. Bouncer does it automatically.
- Laravel doesn’t block spam traps, catch-alls, or toxic emails. Bouncer does.
- Laravel needs coding knowledge to implement validation. Bouncer is no-code-friendly.
- Laravel doesn’t verify deliverability. Bouncer improves inbox placement with real insights.
For businesses that rely on clean, valid email addresses, Bouncer might be a better choice. It ensures your emails reach the right inboxes – technically valid and deliverable.
Conclusion
Before deciding to use Laravel email validation, you should know this – it doesn’t confirm if the email address works. It won’t catch fake emails, spam traps, or risky addresses that can harm your sender reputation. Also, setting up custom validation rules requires coding knowledge, and even then, Laravel won’t verify valid email addresses beyond basic formatting.
Bouncer might be a better alternative. It verifies real emails, blocks spam traps, and automates the entire process – no coding required. Whether you need bulk verification or real-time email checks, Bouncer ensures your emails reach actual inboxes, not dead ends.
Don’t just check if an email looks right – make sure it works. Try Bouncer today with free credits.